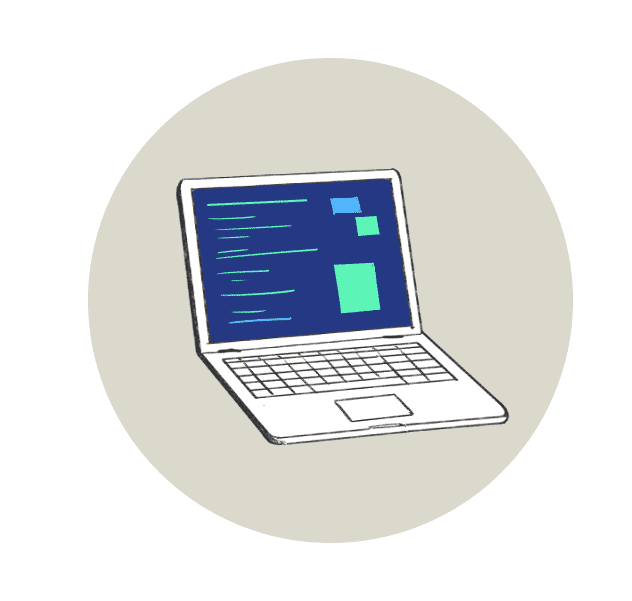
Comments within your code are like icing on a cake. Put too much and it will be overkill, put too little and people will wish they had more. But put the right amount and everyone leaves happy. Of course, the “right amount” depends on the function of your code. We’ve put together five tips to help you comment effectively within your code.
1. Comments are meant for humans
The computer doesn’t read your comments, they are there for you and the other humans who may read it. Simply restating a function or line of code as a sentence within a comment is 1) not valuable and 2) redundant.
Here is an example:
// The code is ready.
var isReady = true;
The comment above is useless because any developer can read that and know that isReady is true. Let’s try something else.
// Default to isReady. Results of function A or function B can change this to false due to XYZ.
// This controls whether ABC happens.
var isReady = true;
That’s a much more useful comment because it fills in the intention behind the code, what can change it, how it relates to other things.
Consider this question before you write a comment: “What would I need to know if I saw this for the first time?”
2. Say why and sometimes what
Read other articles about commenting within code and you’ll see things like “good code explains itself” or “explain why NOT what” and while we agree with this, there are always exceptions to the rule. For example, A brief summary or headline before many lines of code can be helpful for someone skimming through your work or looking for something specific.
Comments are perfect for stating why your code is where it is. If a particular action is causing errors, state what it was and how you worked around it.
NDepend summarized this really well, “Good programmers comment their code. Great programmers tell you why a particular implementation was chosen. Master programmers tell you why other implementations were not chosen.”
Let’s take a look at an example:
// FunctionA seems like it works better here but kept returning errors b/c of Conditions 1 and 2.
// Went with FunctionB() instead
3. Update comments when you update code
Chances are, whatever you are working on will go through multiple iterations. If your code changes, make your comments reflect those changes. The last thing anyone needs is more confusion. Save it for when you can’t find that missing semi-colon or extra parenthesis.
4. Be efficient
Center your efforts around writing strong, readable code instead of OK code with descriptive comments.
Name functions, classes, and IDs effectively and it will be just as good as that descriptive comment you had written before.
Organizing your code goes a long way for readability. The computer reads it all the same so why not make it easier on yourself and the people who may be reading it. Humans like to read in short blocks, not long big blocks of text. Try adding spaces in between unrelated lines to break up long blocks of code.
Take a look at these examples:
Commenting just for the sake of commenting doesn’t help anyone. Let’s go back to our first example:
// The code is ready.
var isReady = true;
The comment above is useless because any developer can read that and know that isReady is true. Let’s try something else.
// Default to isReady. Results of function A or function B can change this to false due to XYZ.
// This controls whether ABC happens.
var isReady = true;
Even though the second comment is longer, it is more descriptive and addresses possible questions a developer may have while looking at it. Now you have saved yourself the time of explaining or answering questions in the future.
5. Be consistent
When deadlines are close and you need to work quickly, it’s tempting to skip the comments and tell yourself you’ll go back later. But let’s be honest, you probably won’t go back later so comment as you go. The reasons for leaving comments or documentation will be fresh in your head.
Humans love patterns. Once you’ve picked a convention that works for you like breaking up your code into related blocks in the beginning, stick with it.
Comments should be put in place to make life easier, not harder. Whether you’re a junior developer or code jedi, these five tips should help you keep your comments fresh and effective.